Step 1
First you need to copy the KoolControls folder onto your web server/localhost.Step 2
Create an index.php file in on the same level with the KoolControls folder, open the file and include the KoolGrid and KoolAjax php files: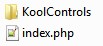
include "KoolControls/KoolGrid/koolgrid.php";
include "KoolControls/KoolAjax/koolajax.php";
Step 3
Create a new grid, point its scriptFolder the KoolGrid folder and set its style::$grid = new KoolGrid("grid");
$grid->scriptFolder = "KoolControls/KoolGrid";
Step 4
Create a new connection, then a data source with a select command and set it for the grid:$db_con = mysql_connect($dbhost,$dbuser,$dbpass);
mysql_select_db($dbname,$db_con);
$ds = new MySQLDataSource($db_con);
$ds->SelectCommand = "select customerNumber,customerName,phone,city from customers";
$grid->DataSource = $ds;
Step 5
Set up some properties for the grid:$grid->AjaxEnabled = true; //Enable operating the grid with ajax.
$grid->Width = "655px";
$grid->AllowInserting = true;
$grid->AllowSorting = true;
$grid->AutoGenerateColumns = true; //Let the grid auto create columns based on the select command for you.
$grid->MasterTable->Pager = new GridPrevNextAndNumericPager(); //Create a kind of pager for the grid. There're other kinds for your choice.
Step 6
Finally process and render the grid:<?php $grid->Process();?>
<body>
<form id="form1" method="post"> <!-- You should render the grid inside a form tag, in case $grid->AjaxEnabled = false the grid needs a form to operate. -->
<?php echo $koolajax->Render();?>
<?php echo $grid->Render();?>
</form>
</body>
And that's it. Here's our resulted index.php file:
<?php
include "KoolControls/KoolGrid/koolgrid.php";
include "KoolControls/KoolAjax/koolajax.php";
$grid = new KoolGrid("grid");
$grid->scriptFolder = "KoolControls/KoolGrid";
$db_con = mysql_connect($dbhost,$dbuser,$dbpass);
mysql_select_db($dbname,$db_con);
$ds = new MySQLDataSource($db_con);
$ds->SelectCommand = "select customerNumber,customerName,phone,city from customers";
$grid->DataSource = $ds;
$grid->AjaxEnabled = true; //Enable operating the grid with ajax.
$grid->Width = "655px";
$grid->AllowInserting = true;
$grid->AllowSorting = true;
$grid->AutoGenerateColumns = true; //Let the grid auto create columns based on the select command for you.
$grid->MasterTable->Pager = new GridPrevNextAndNumericPager(); //Create a kind of pager for the grid. There're other kinds for your choice.
$grid->Process();
?>
<body>
<form id="form1" method="post"> <!-- You should render the grid inside a form tag, in case $grid->AjaxEnabled = false the grid needs a form to operate. -->
<?php echo $koolajax->Render();?>
<?php echo $grid->Render();?>
</form>
</body>
You could go to this online demo to see the example.php code below the page:
KoolGrid example
A few notes:
- In the example.php code, a database connection was created in the file Resources/runexample.php of our KoolPHPSuite package.
- For types of database other than MySQL, please include the relevant data source class located in the folder "KoolControls/KoolGrid/ext/datasources". We support the most popular database connections including: Oracle, MS SQL Server, MySQL, PostgreSQL, PDO, ODBC, Firebird.
- If you want to manually create your columns (there are various types), please go to this demo and documentation: Demo -- Documentation